Cookie – PHP Advanced
Its a small file that server insert on user’s computer. When the same computer requests a page with a browser, it will send cookie too. You can create and retrieve cookie values here in PHP.
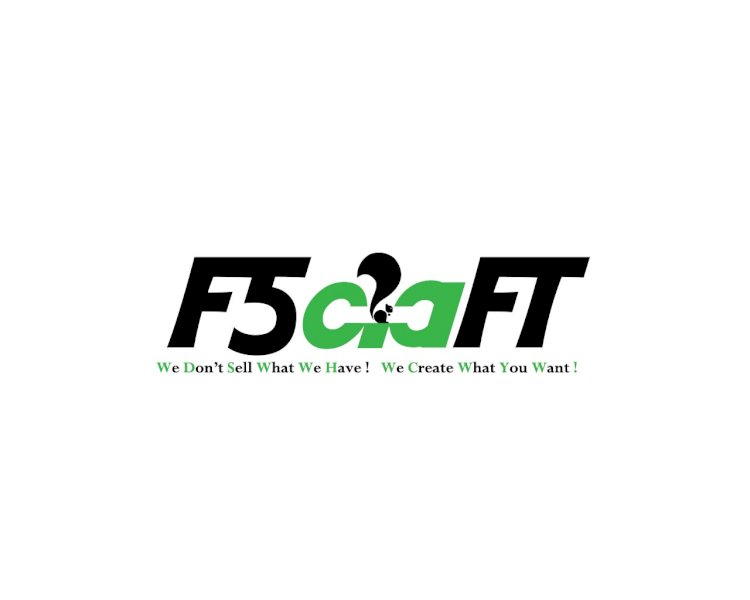
Its a small file that server insert on user’s computer. When the same computer requests a page with a browser, it will send cookie too. You can create and retrieve cookie values here in PHP.
Create Cookies
setcookie() function is used to create a cookie.
//cookies syntax setcookie(name, value, expire, path, domain, secure, httponly);
Following code creates a cookie named “cust” with value “Code Projects” & after 30 days cookie will expire. “/” means that cookie is available in entire website.
<!DOCTYPE html> <?php $cookie_name = "cust"; $cookie_value = "Code Projects"; setcookie($cookie_name, $cookie_value, time() + (86400 * 30), "/"); // 1 day = 86400 ?> <html> <body> <?php if(!isset($_COOKIE[$cookie_name])) { echo "Cookie Named '" . $cookie_name . "' is not set!"; } else { echo "Cookie '" . $cookie_name . "' is set!<br>"; echo "Value is: " . $_COOKIE[$cookie_name]; } ?> <p><b>NOTE:</b> To see the value of cookie RELOAD the page if value of cookie is not shown.</p> </body> </html>
Output :
Modifying Cookie Value
To modify cookie value just set the value again.
<!DOCTYPE html> <?php $cookie_name = "cust"; $cookie_value = "Johnny"; setcookie($cookie_name, $cookie_value, time() + (86400 * 30), "/"); ?> <html> <body> <?php if(!isset($_COOKIE[$cookie_name])) { echo "Cookie Named '" . $cookie_name . "' is not set!"; } else { echo "Cookie '" . $cookie_name . "' is set!<br>"; echo "Value is: " . $_COOKIE[$cookie_name]; } ?> <p><b>Note:</b> To see the value of cookie RELOAD the page if value of cookie is not shown.</p> </body> </html>
Output :
Deleting Cookie
Use setcookie( ) function with past expiration date to delete cookie
<!DOCTYPE html> <?php // set the expiration date to one hour ago setcookie("user", "", time() - 3600); ?> <html> <body> <?php echo "Cookie 'user' is deleted."; ?> </body> </html>
Output :
Check if Cookies are Enabled
<!DOCTYPE html> <?php setcookie("ex_cookie", "test", time() + 3600, '/'); ?> <html> <body> <?php if(count($_COOKIE) > 0) { echo "Cookies are enabled."; } else { echo "Cookies are disabled."; } ?> </body> </html>
Output :
MessengerWhatsApp