How to unzip or extract zip file using PHP
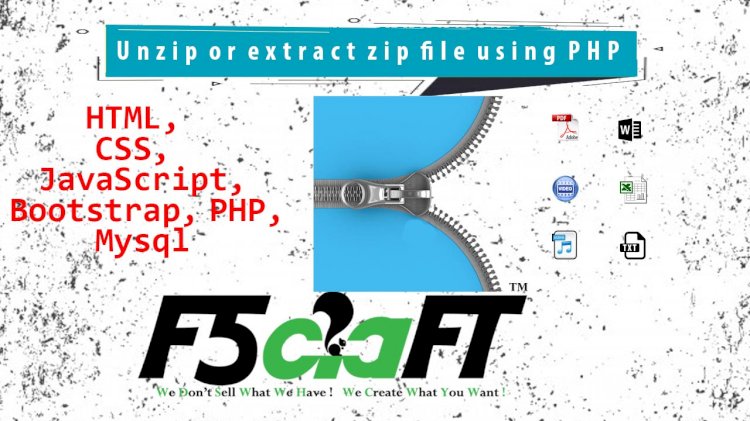
Here is the simple code which will help you to unzip or extract zip file using php. In this tutorial we are using ZipArchive class of php to do so. ZipArchive is a built in class of PHP. ZipArchive was introduced in PHP version 5.2. So, your PHP version should be 5.2 or greater. So friends, in this tutorial you will see how to unzip or extract zip file using PHP. Please visit the following link which will show you how to create a zip file using php.
How to unzip or extract zip file using php
Now use the below code to unzip or extract zip file.
- <?php
- //Check whether zip extension is enabled or not
- if(extension_loaded('zip')) {
- $zip = new ZipArchive();
- //Path of the source zip file to be extracted
- $source = "test.zip";
- if ($zip->open($source) === TRUE) {
- //Destination of extracted files and folders to be stored
- $destination = "extracted/";
- $zip->extractTo($destination);
- $zip->close();
- } else {
- echo "Failed to open the zip file!";
- }
- }
- ?>
- <?php
- //First check whether zip extension is enabled or not
- if(extension_loaded('zip')) {
- $zip = new ZipArchive();
- ?>
Check whether the zip extension is enabled or not. If yes, then create an object of the ZipArchive class. This object will help you to call various default functions of the ZipArchive class.
- <?php
- if($zip->open($source) === TRUE) {
- ----------------------
- ----------------------
- } else {
- echo "Failed to open the zip file!";
- }
- ?>
If the source zip file is not found it will simply print a message that “Failed to open the zip file!”.
- <?php
- //Destination of extracted files and folders to be stored
- $destination = "extracted/";
- $zip->extractTo($destination);
- $zip->close();
- ?>
$zip->extractTo() function extracts zip file and stores all the files and folders of it in the predefined folder. $zip->close() simply close and free the resource.
Here is the complete code of the article “How to unzip or extract zip file using php” —
- <?php
- if(isset($_POST['extractZip'])) {
- //Check whether zip extension is enabled or not
- if(extension_loaded('zip')) {
- $zip = new ZipArchive();
- //Path of the source zip file to be extracted
- $source = "test.zip";
- if ($zip->open($source) === TRUE) {
- //Destination of extracted files and folders to be stored
- $destination = "extracted/";
- $zip->extractTo($destination);
- $zip->close();
- } else {
- echo "Failed to open the zip file!";
- }
- } else {
- echo "Zip extension is not enabled!";
- }
- }
- ?>
- <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
- <html xmlns="http://www.w3.org/1999/xhtml">
- <head>
- <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" />
- <title>How to extract or unzip a zip file using PHP || Mitrajit's Tech Blog</title>
- <style>
- span { clear:both; display:block; margin-bottom:30px; }
- span a { font-weight:bold; color:#0099FF; }
- table { margin-top:30px; }
- .msg-div { margin-top:20px; }
- ul { margin:0; padding:0; }
- ul li { list-style:none; margin:10px; }
- img { margin-right:10px; }
- h3 { border-bottom:2px solid #000; }
- </style>
- </head>
- <body>
- <span>Read the full article -- <a href="http://www.mitrajit.com/2016/10/unzip-extract-zip-file-using-php/" target="_blank">How to unzip or extract zip file using PHP</a> in Mitrajit's Tech Blog</span>
- <form action="" method="post">
- <input type="submit" name="extractZip" value="Extract Zip" />
- </form>
- <?php
- if(isset($_POST['extractZip'])) {
- echo "<h3>Extracted files and folders</h3>";
- echo "<ul>";
- foreach(glob('extracted/*') as $filename)
- {
- echo "<li>";
- $filenames = explode("/", $filename);
- if(is_dir($filename)) echo "<img src='images/folder.gif' width='25' height='25' align='absmiddle'>".$filenames[1];
- else echo "<img src='images/file.gif' width='25' height='25' align='absmiddle'>".$filenames[1];
- echo "</li>";
- }
- echo "</ul>";
- }
- ?>
- </body>
- </html>
If you enjoy this article and think it is useful then please share this article with others.
Need a Website Or Web Application Or Any Help In Code , Contact Us: +91 8778409644 (Whatsapp) or Email: uma@f5craft.com | Visit: www.f5craft.in /.com, Note: Paid Service Only